Vim & Rust
Sounds like the title of a thrash metal album doesn't it?
Well this post won't melt your speakers, but it may make your editor scream with joy as I'm going to go through how I have set up vim for development with rust! Previously I have walked through building vim entirely from source, installing a plugin manager alongside YouCompleteMe and tweaking the vimrc with some keybindings. Today's post will build on that, where we enable rust language support inside YCM, adding more plugins and tweaking the vimrc further.
Rust & YCM
You may already know that YCM natively supports C, C++, Python, Typescript, Java etc. But what you might not know is that it also supports Rust! YCM is a language server protocol client; thus it enables another server to communicate to it, unlocking support for intelligent code completion, syntax checking, diagnostics and error checking which is fantastic for us as we can harness the power of rust-analyzer inside of vim. This powers-up vim further to be a fair combatant in the world of IDE's in 2025.
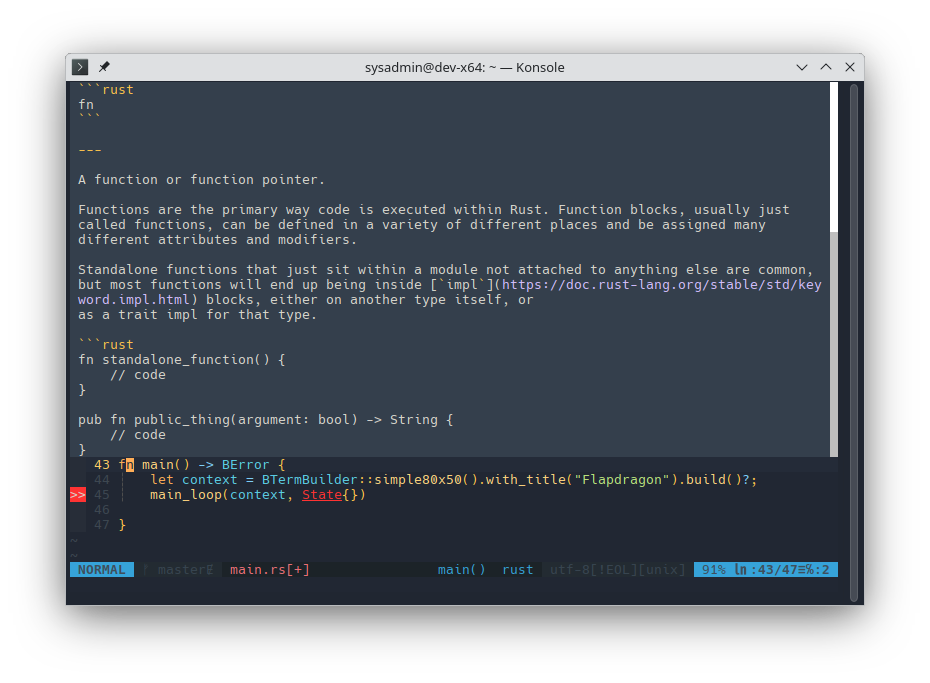
YCM in action, working with rust-analyzer. In Vim's normal mode, highlighting keywords in the syntax will bring up detailed popups. I keep them on, but they can be disabled in the vimrc as seen below. I'm currently working through a Rust game development book; if you are interested about the code itself.
Adding support for YCM
If you followed the previous blog post you will be ready to go with installing the support needed, which is extremely easy to do. If you didn't follow the previous post, I strongly suggest you do so because setting up YCM is no mean feat and the previous post will cover all prerequisites. Then; we simply need to tell YCM where to look for the rust analyzer and how can we do that? the vimrc. You betcha! simply add these lines to it, replacing your-username with your systems account username.
let g:ycm_language_server = [
\ {
\ 'name': 'rust',
\ 'cmdline': [ '/home/your-username/.cargo/bin/rust-analyzer' ],
\ 'filetypes': [ 'rust' ],
\ }
\ ]
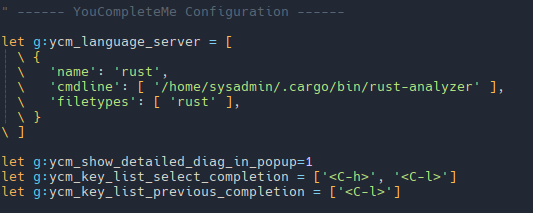
A snippet from my personal vimrc, showing the config I use for YCM. Note the setting for detailed dialog popups, they can be disabled by setting the value to 0.
After editing, cd into a rust project, open the src/main.rs in vim and rust-analyzer will be ready to go. write some simple code, such as a function block and watch the magic unfold! You can usually force an auto-completion with CTRL+SPACE or CTRL+N. These keybinds can be remapped; as we have already found out through the power of vimrc.
Enabling Clippy
Alongside rust-analzer and YCM, if you're a "Dark Souls" coder and love being punished, you can enable Clippy in your projects. Clippy will monitor your code and ~gently~ remind you when you are falling foul of code guidelines. Punish yourself further and enable pedantic mode if you really want Clippy to be brutal with your code, it's a great way of ensuring your projects are up to code guidelines and your coding style is not picking up bad habits. You can either run clippy directly from your projects directory with cargo clippy
, or add this line to the top of your main.rs to enable Clippy:
#![warn(clippy::all)]
To enable pedantic mode, use this:
#![warn(clippy::all, clippy::pedantic)]
You might get frustrated at using it at first as it is pretty brutal regarding rust code, but let it help you. Clippy is a valuable tool for teaching you how to write great code.
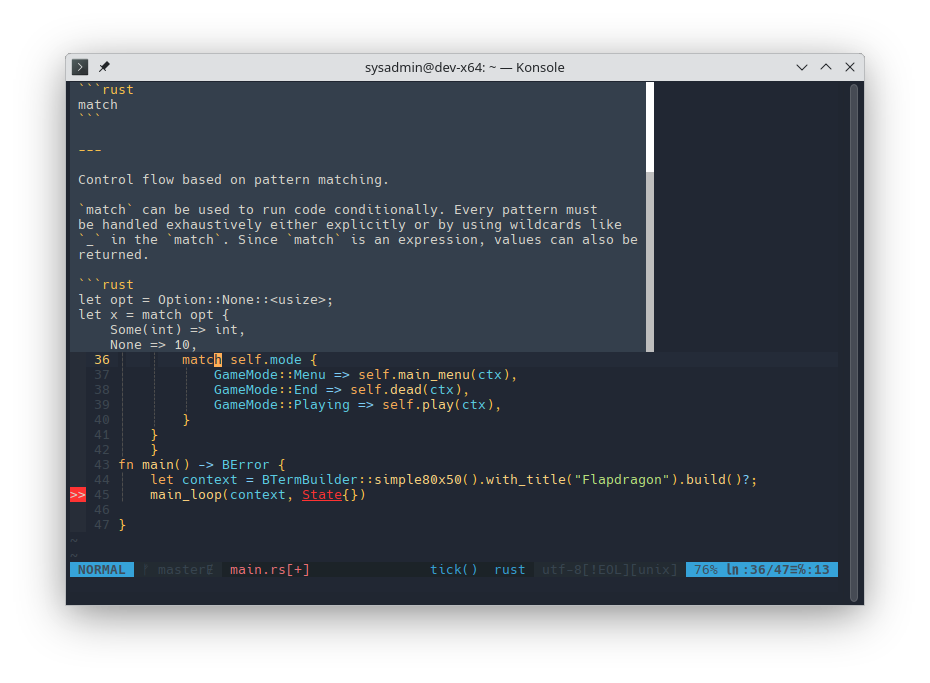
YCM in action, showing a detailed description of "match"
Rust Plugins
There are a few rust plugins available for vim:
rust-lang/rust.vim - Rust Developer Essential. This plugin adds essential features: syntax highlighting, code formatting through rustfmt, and integration with the Rust Language Server.
timonv/vim-cargo - Adds cargo support directly to vim, enabing project building, testing and running straight from the editor. Typing :CargoBuild
, :CargoTest
or :CargoRun
will build, test or run your project directly from vim's command mode.
arzg/vim-rust-syntax-ext - This nifty little plugin enhances Vim's Rust syntax abilities, providing extra syntax rules and refinements. Makes Rust code more readable inside of vim.
A few other non-rust plugins I will mention to improve your vim setup are:
preservim/nerdtree - NERDTree File browser, enables a file explorer inside vim.
preservim/tagbar - TagBar, generates tags based on your code showing functions, classes, variables, etc. Think of it as a table of contents for your code.
vim-airline/vim-airline - A beautiful statusline for vim, shows the current editing mode, encoding, cursor position and renders tabs beautifully. Highly configurable.
vim-airline/vim-airline-themes - Automatically matches airline with a visually appealing theme to compliment the theme you are using.
Add these into your vimrc, run :PluginInstall (you should be an expert at this by now!) and enjoy the power these plugins add to your vim setup.
Extending it further
We can extend our vim setup further with git integration, absolutely essential these days and luckily for us, tpope/vim-fugitive is perfect for this. Not only supporting git, but github also enabling you to manage your repo's all in the comfort of vim's command mode:
:G status - View the current status of your repository.
:G diff - Compare changes in the current file with the last commit.
:G commit - Stage changes and commit them, all from within Vim.
:G push & :G pull - Push and pull changes to/from your remote repository.
:G log - View git log
:GBrowse - opens the current file directly in your GH repo via your web browser. Extremely useful for sharing code to a friend or team-mate.
There are more commands, if you're interested in diving further check out tpope's fugitive repository.
Going even further than that; we can even install airblade/vim-gitgutter to monitor files in real-time without running git diff. This enables you to check for modifications in your code, such as added or removed lines, all within vim.
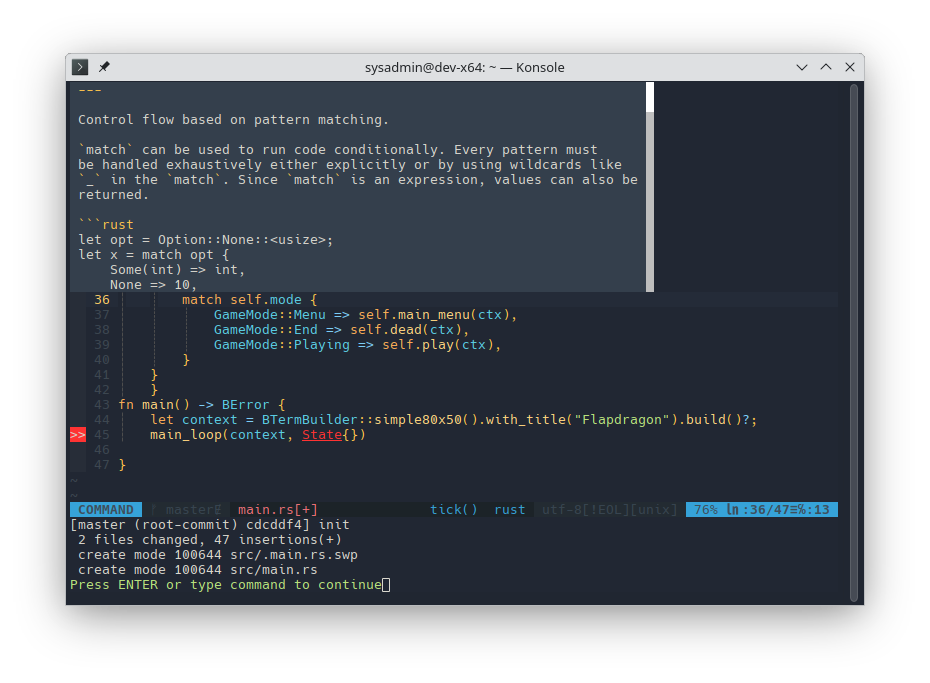
fugitive at work, showing a commit made entirely inside Vim with
:G commit -m "init"
You should have a pretty decent vim setup by now. We can extend it even further with themes (you could even create your own), however themes are a very personal matter and you will have to find the one that "speaks" to you. The same applies to keybindings, I could go on at length about keybindings, but these are also a personal thing and aren't generic. For instance I have "jj" remapped in vim to "esc" to escape normal mode without my fingers over-reaching and leaving the modal/vim keys (sdfg + hjkl), but I also have other keybindings that won't work unless you have a keyboard that has layers. You should be a .vimrc expert by now and remapping keys inside the vimrc shouldn't be a difficult task for you.
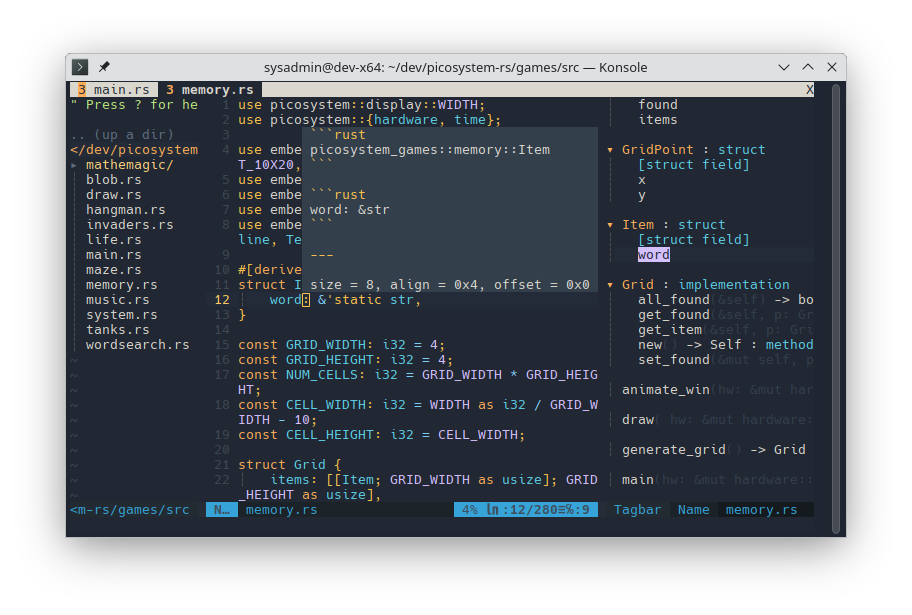
Vim, Rust & YCM working together in harmony. Tabbed workspaces, NERDTree and TagBar, showing the beauty of ayu-mirage theme and vim-rust-syntax-ext's extra syntax capabilities.
Final thoughts
Well that's it. Vim & Rust. Let's quickly cover the features we've added:
YCM & rust-analyzer - intelligent code completion
Clippy - code syntax/guideline helper
Rust Plugins - Essential plugins for Rust
fugitive & gitgutter - git integration and real-time git diff
That's a pretty awesome Vim setup for Rust if you ask me. I hope this post was helpful and enabled you to install support for Rust inside of Vim. Now get coding!
Catch you next time,
Dru x